Salesforce’s Lightning Web Components (LWC) is a popular framework for developing web applications on the Salesforce platform. LWC offers several decorators, including track
, wire
, and api
, that developers use to extend the functionality of their components. In this post, we’ll dive into the differences between these three decorators.
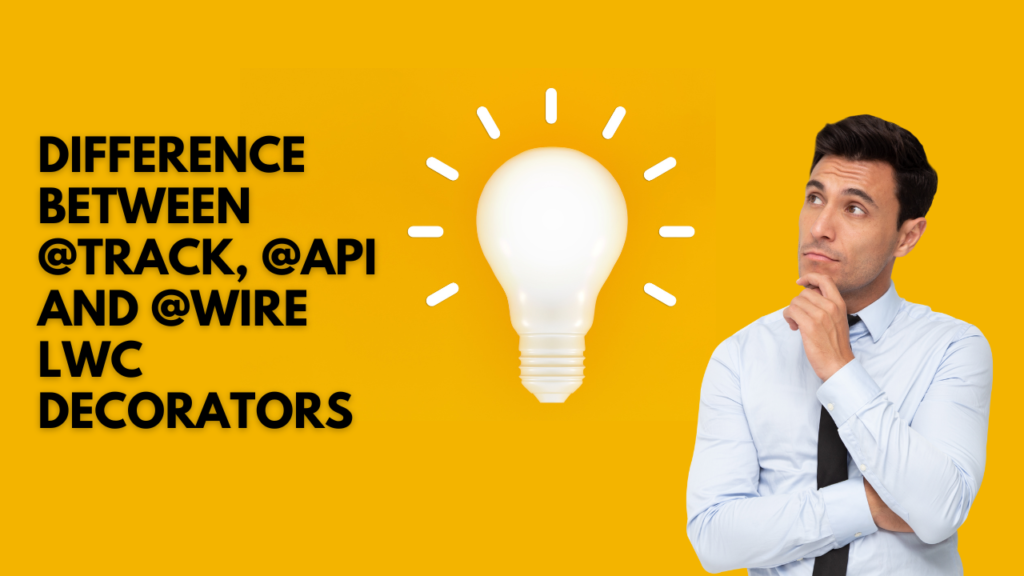
Track Decorator
The track
decorator is used to track changes to a property’s value within a component. Whenever the value of a tracked property changes, the component rerenders, reflecting the updated value.
import { LightningElement, track } from 'lwc';
export default class ExampleComponent extends LightningElement {
@track greeting = 'Hello';
handleChange(event) {
this.greeting = event.target.value;
}
}
Wire Decorator
The wire
decorator is used to retrieve data from server-side controllers or Apex methods. The data returned by the method is stored in the component’s property, and the component rerenders with the new data.
import { LightningElement, wire } from 'lwc';
import getContacts from '@salesforce/apex/ContactController.getContacts';
export default class ContactList extends LightningElement {
@wire(getContacts) contacts;
}
API Decorator
The api
decorator is used to expose a component’s property or method to the parent component. This allows the parent component to access the property or method.
import { LightningElement, api } from 'lwc';
export default class ExampleComponent extends LightningElement {
@api message = 'Hello World';
}
Summary
In summary, the track
decorator is used to track changes to a property’s value within a component, the wire
decorator is used to retrieve data from server-side controllers or Apex methods, and the api
decorator is used to expose a component’s property or method to the parent component. These decorators are powerful tools for building robust and scalable web applications on the Salesforce platform.
Leave a Reply