Salesforce provides developers with a number of different ways to call Apex methods from Lightning Web Components (LWC). In this blog post, we’ll explore these different methods and their advantages and disadvantages, along with Apex code examples to help illustrate the concepts.
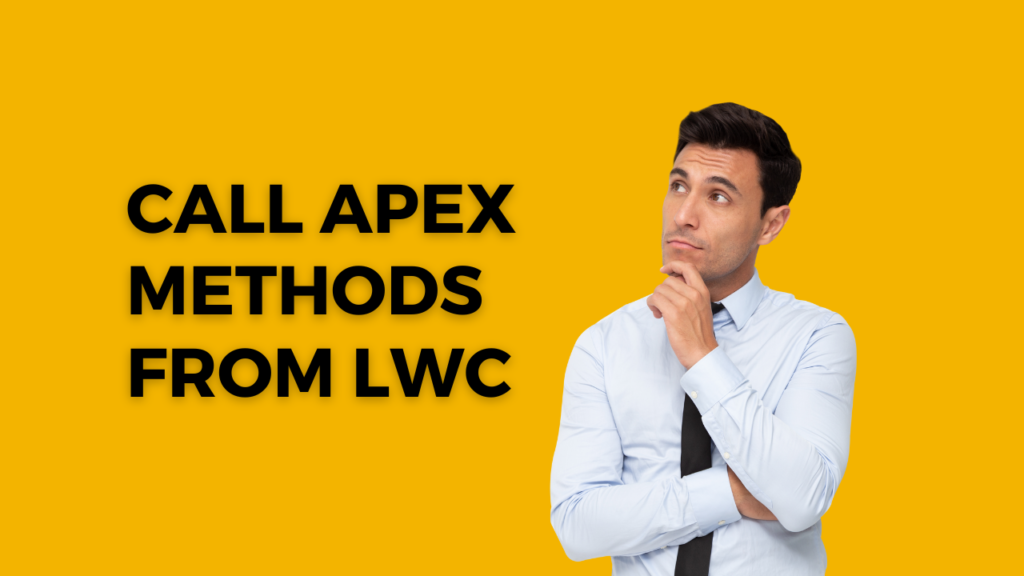
1. Calling Apex Methods Using @wire
Advantages:
- Automatic handling of data retrieval and data update in the component.
- Code is concise and easy to understand.
Disadvantages:
- Limited to only one Apex method call per wire decorator.
- Cannot perform complex business logic in the component.
The @wire decorator is a powerful tool for fetching data from Apex methods. With @wire, the component is automatically updated whenever the underlying data source changes. Here’s an example of how to use @wire to call an Apex method:
import { LightningElement, wire } from 'lwc';
import getContacts from '@salesforce/apex/ContactController.getContacts';
export default class ContactList extends LightningElement {
@wire(getContacts) contacts;
}
2. Calling Apex Methods Imperatively
Advantages:
- Ability to call multiple Apex methods in a single component.
- More control over the Apex method calls.
Disadvantages:
- No automatic handling of data retrieval and data update in the component.
- Code can become more verbose and difficult to understand.
Imperative Apex method calls provide more control over the flow of the code, allowing developers to perform complex business logic in the component. Here’s an example of how to use imperative Apex method calls:
import { LightningElement } from 'lwc';
import getContacts from '@salesforce/apex/ContactController.getContacts';
export default class ContactList extends LightningElement {
contacts;
connectedCallback() {
getContacts()
.then(result => {
this.contacts = result;
})
.catch(error => {
console.error(error);
});
}
}
3. Calling Apex Methods with Parameters
Advantages:
- Ability to pass parameters to the Apex method call.
- More flexible code.
Disadvantages:
- No major disadvantages.
Passing parameters to Apex method calls is a common requirement in many applications. Here’s an example of how to call an Apex method with parameters using both the @wire decorator and the imperative approach:
import { LightningElement, wire } from 'lwc';
import getContactsByAccountId from '@salesforce/apex/ContactController.getContactsByAccountId';
import { getRecord } from 'lightning/uiRecordApi';
export default class ContactList extends LightningElement {
accountId;
contacts;
@wire(getRecord, { recordId: '$accountId', fields: ['Account.Name'] })
account;
handleAccountIdChange(event) {
this.accountId = event.target.value;
}
@wire(getContactsByAccountId, { accountId: '$accountId' })
wiredContacts({ error, data }) {
if (data) {
this.contacts = data;
} else if (error) {
console.error(error);
}
}
get hasResults() {
return this.contacts && this.contacts.length > 0;
}
}
Conclusion
Calling Apex methods from LWC in Salesforce is a common requirement for many Lightning applications. Depending on the specific use case, there are different methods to choose from, each with its own advantages and disadvantages.
The @wire decorator is the recommended approach for most situations, as it provides automatic handling of data retrieval and data update in the component. Imperative Apex method calls and Apex method calls with parameters offer more flexibility and control, but can be more verbose and difficult to understand.
By understanding the strengths and weaknesses of these different methods, developers can choose the best approach for their specific use case, leading to more efficient and effective code.
Leave a Reply