If you are new to Salesforce, this topic is for you. Throughout your career, you will definitely have a requirement to integrate with at least one of the third party system either using REST or SOAP API. Here I will show you a simple, “Hello Word” program to send the request to endpoint.
To begin with, we will be using the website https://requestbin.com/ for testing our integration requests.
Steps to follow:
- First thing is to create an endpoint. Visit Requestbin and click on “Create Request Bin“.
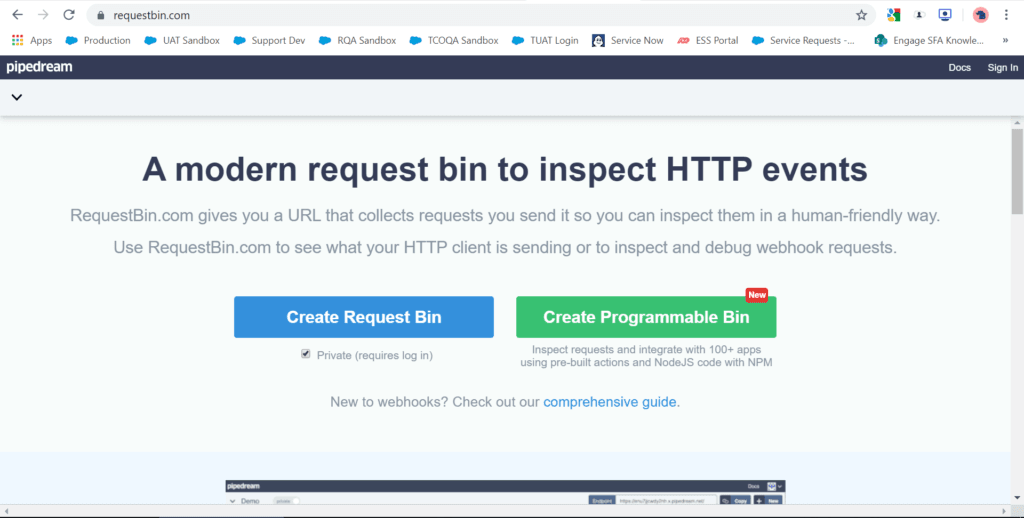
- Choose either if you want to login via Github or Google account. If you are new user, it will ask you to put username to create an account.
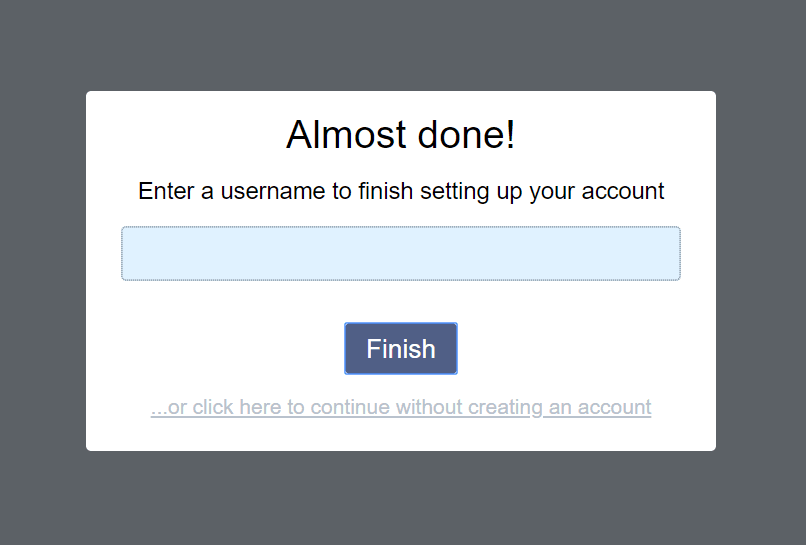
- Once you landed on dashboard, you will see a link. This is the link we need to use to send the data. On right sidebar you can see the its continuously checking if any incoming request is coming. Even if you open this URL in new tab, it will capture your request.
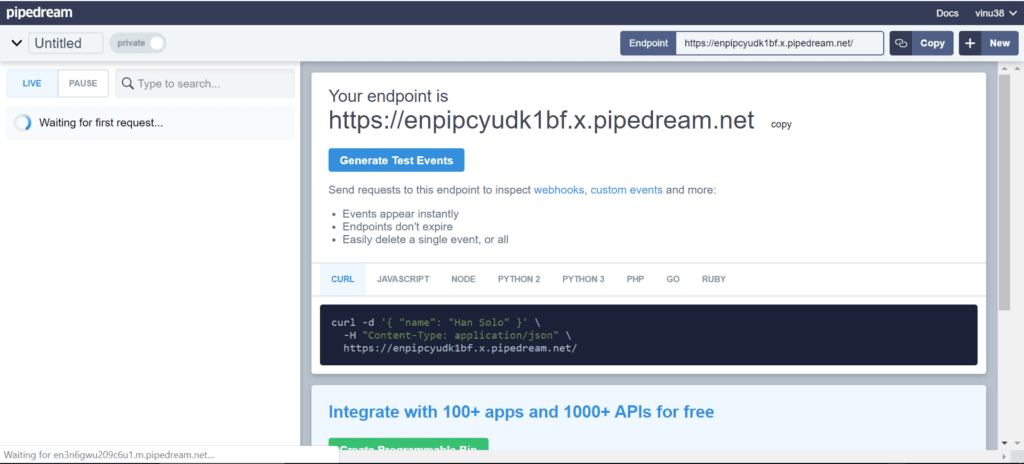
- We are done with endpoint setup, its time to configure in Salesforce. Before having any callout, Salesforce want us to white list the endpoint to prevent any unauthorized callouts. To do that, login to your Salesforce org, navigate to Setup -> Remote Site Settings.
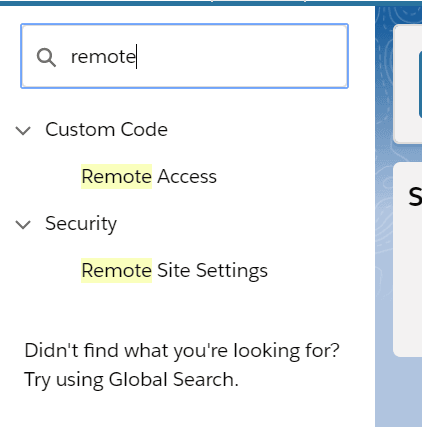
- Click on “New Remote Site”, Put following information:
- Remote Site Name : Test_Callout
- Remote Site URL : Put the generated URL from RequestBin
- Disable Protocol Security : Uncheck (Check if your URL is not secure)
- Description : Test
- Active : Check
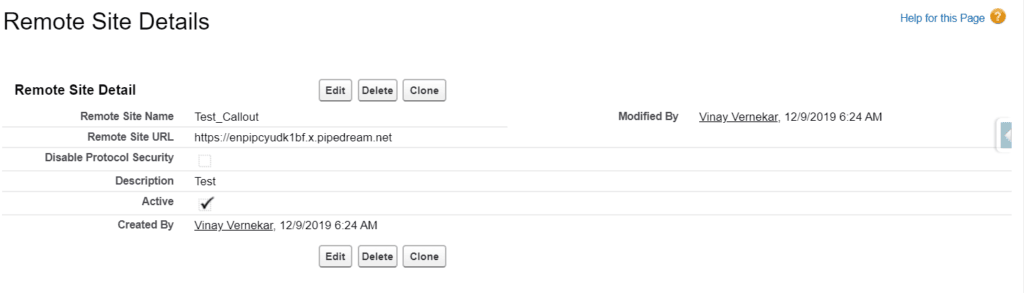
- Time to create an apex class. Open developer console and create new class “CalloutUtility“. Copy and paste following code in class and save it.
- Endpoint : REST API url to where you want to send the request
- Method : GET, POST, DELETE, PUT
- Headers : Content-type, Authentication information etc.
- Body : JSON, XML, Text
- TimeOut : Maximum of 120 seconds, if server doesn’t respond within specified timeout, callout will fail.
/***********************************************
* Name : CalloutUtility
* Developer: Vinay Vernekar
* Website: https://sfdcdevelopers.com
* Purpose: Utility class for callouts
* **********************************************/
public class CalloutUtility {
public static HTTPResponse doCallout(string EndPoint, string Method, map<string,string> Headers, string Body, integer TimeOut){
HttpRequest req = new HttpRequest();
req.setEndpoint(EndPoint);
req.setMethod(Method);
if(Body != '')
req.setbody(body);
req.setTimeout(TimeOut);
for(String HeaderKey : Headers.keySet())
req.setHeader(HeaderKey, Headers.get(Headerkey));
Http http = new Http();
HTTPResponse response = http.send(req);
return response;
}
}
- Its always a best practice to have a Utility class which will perform specific task. Here we created Utility class for callouts, so whenever you need to perform REST callout, you can call the doCallout method from CalloutUtility class.
- Now how to test it? Open execute anonymous window and execute following code:
CalloutUtility.doCallout('URL_From_RequestBin', 'POST', new map<string, string>{'content-type' => 'application/json'}, '{"URL" : "SFDCDevelopers.com"}', 120000);
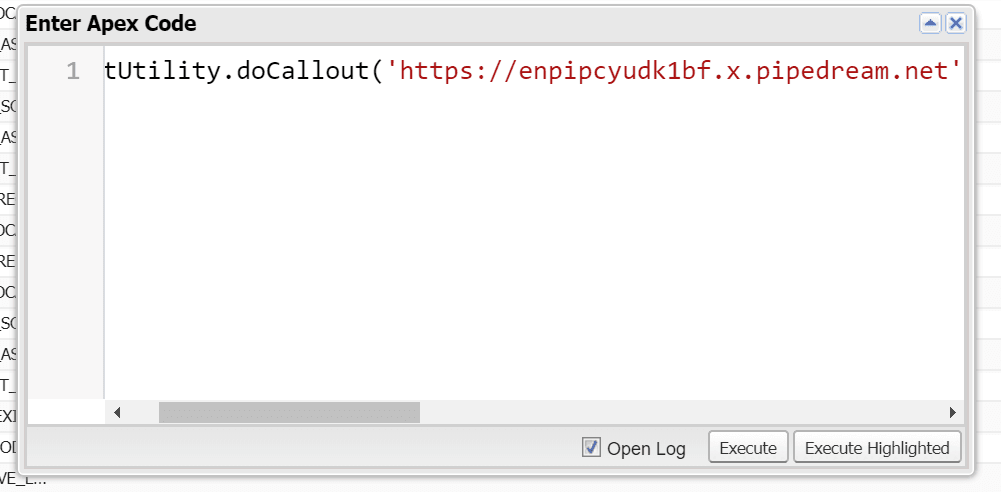
- Replace “URL_From_RequestBin” with the URL of RequestBin. Here in this request we specifying the request we are doing is of JSON type by passing header as ‘content-type’ => ‘application/json’. Body is {“URL” : “SFDCDevelopers.com”} in JSON format. Timeout is 120 seconds.
- Make sure to check “Open Log” checkbox. Not click on “Execute”.
- If you open RequestBin window now, you can see one request has been received with the data we sent.
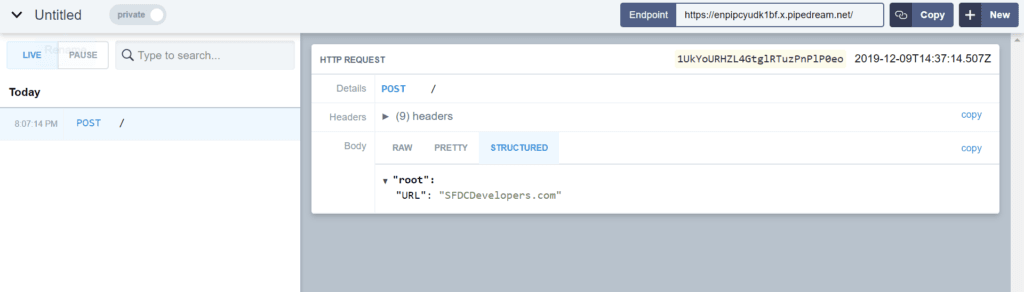
- Also in the debug logs, you can see the Status of callout.
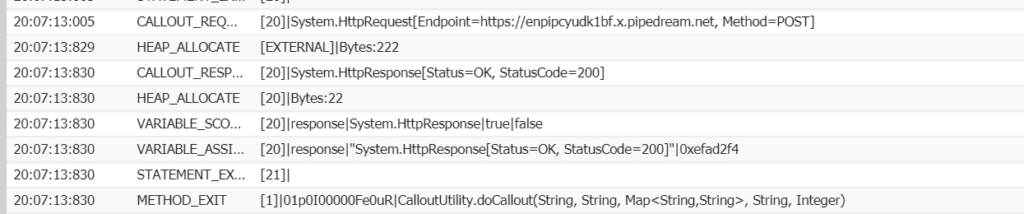
- You can extend the logic further to perform additional functionality. We will be covering some advanced topics near in future.
Leave a Reply