Most of you may think to show the list of child records of a parent record on visualforce page standard controller, apex extension is required. However this does not require any extension class. I will explain you how you can do this:
Problem Statement
Create an inline visualforce page to display the list of “Active” contacts under an account.
Approaches
As this is a inline visualforce page, standard controller is must. There are two approaches to build this:
- Create an apex extension to query all “Active” (Status is a custom field created on contact) contacts
- Show list with the help of child relation
Here in this post, I will show you the how second approach will work. The visualforce page code as follows:
<apex:page standardController="Account">
<apex:pageBlock title="{!Account.Name+' Active Contacts'}">
<Apex:pageBlockTable value="{!Account.Contacts}" var="con">
<Apex:column rendered="{!con.Status__c = 'Active'}">
{!Con.FirstName}
</apex:column>
<Apex:column rendered="{!con.Status__c = 'Active'}">
{!Con.LastName}
</apex:column>
<Apex:column rendered="{!con.Status__c = 'Active'}">
{!Con.Email}
</apex:column>
<Apex:column rendered="{!con.Status__c = 'Active'}">
{!Con.Phone}
</apex:column>
</Apex:pageBlockTable>
</apex:pageBlock>
</apex:page>
Assumptions
- Created custom field “Status” on contact with values as
- Active
- Inactive
Explanation
- Apex:pageBlockTable : To show table of contact records.
- value=”{!Account.Contacts}” : This is important. Contacts is a child relationship API name. It’s referring to all contacts of an Account. You can use any child relationship API name here. For custom object relationship, you can access it with “__r”.
- Apex:column : To show the columns, referring each to field of contact.
- rendered=”{!con.Status__c = ‘Active’}” : Condition to show only Active contacts.
Output
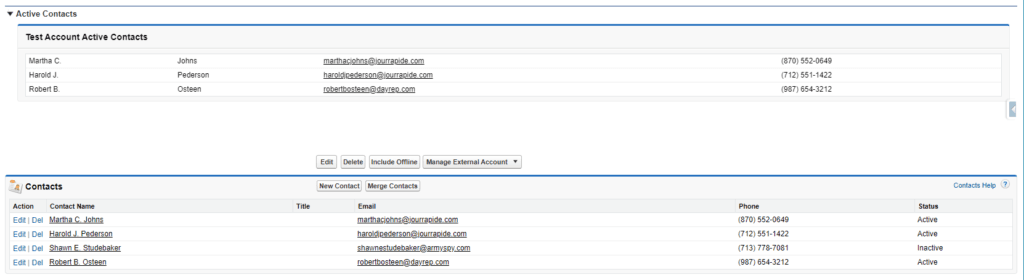
As you can see, first section is inline visualforce page that we developed, showing all active contacts only. In second section, its a contact related list having total 4 contacts, out from which, one contact is inactive.
Leave a Reply